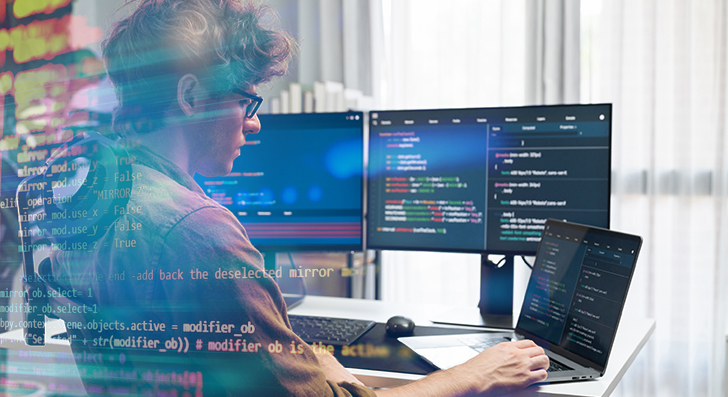
Scalability signifies your software can handle advancement—additional consumers, much more details, plus more targeted visitors—without the need of breaking. For a developer, creating with scalability in mind will save time and anxiety later. Right here’s a transparent and functional guidebook to help you get started by Gustavo Woltmann.
Layout for Scalability from the Start
Scalability just isn't one thing you bolt on later on—it ought to be portion of your system from the beginning. Lots of programs are unsuccessful after they grow fast due to the fact the original layout can’t deal with the additional load. As a developer, you must Believe early regarding how your technique will behave under pressure.
Start out by creating your architecture being flexible. Prevent monolithic codebases exactly where almost everything is tightly related. As a substitute, use modular design or microservices. These designs crack your application into smaller sized, impartial parts. Every single module or company can scale on its own without impacting The complete method.
Also, think of your databases from working day a single. Will it need to have to take care of one million people or simply just a hundred? Choose the proper form—relational or NoSQL—based on how your information will expand. Prepare for sharding, indexing, and backups early, Even when you don’t will need them still.
A further crucial position is to stay away from hardcoding assumptions. Don’t generate code that only works under present situations. Think of what would come about If the person foundation doubled tomorrow. Would your app crash? Would the database slow down?
Use style and design styles that guidance scaling, like concept queues or celebration-pushed programs. These support your app manage a lot more requests without having finding overloaded.
Any time you Make with scalability in your mind, you're not just preparing for success—you might be cutting down long run complications. A perfectly-prepared technique is easier to maintain, adapt, and mature. It’s superior to get ready early than to rebuild later.
Use the Right Databases
Deciding on the ideal databases is actually a important part of making scalable apps. Not all databases are constructed the same, and utilizing the Improper one can slow you down or maybe lead to failures as your app grows.
Start out by comprehension your information. Can it be very structured, like rows in a desk? If Of course, a relational database like PostgreSQL or MySQL is a superb suit. They are potent with associations, transactions, and regularity. Additionally they support scaling tactics like study replicas, indexing, and partitioning to handle far more visitors and facts.
Should your details is much more adaptable—like user action logs, product catalogs, or paperwork—contemplate a NoSQL possibility like MongoDB, Cassandra, or DynamoDB. NoSQL databases are far better at managing big volumes of unstructured or semi-structured knowledge and will scale horizontally far more conveniently.
Also, contemplate your examine and create styles. Are you currently executing lots of reads with less writes? Use caching and browse replicas. Are you presently handling a weighty generate load? Consider databases that could deal with substantial produce throughput, or even occasion-dependent details storage systems like Apache Kafka (for short-term knowledge streams).
It’s also clever to Imagine ahead. You might not have to have Sophisticated scaling characteristics now, but picking a databases that supports them usually means you received’t need to switch later.
Use indexing to speed up queries. Steer clear of avoidable joins. Normalize or denormalize your data based on your access patterns. And usually keep track of database efficiency as you expand.
In brief, the correct databases will depend on your application’s framework, pace demands, And just how you count on it to expand. Get time to pick wisely—it’ll save a great deal of difficulties later on.
Optimize Code and Queries
Fast code is essential to scalability. As your application grows, every single modest delay adds up. Improperly published code or unoptimized queries can decelerate general performance and overload your procedure. That’s why it’s essential to Create productive logic from the start.
Get started by producing clear, straightforward code. Steer clear of repeating logic and take away nearly anything avoidable. Don’t select the most complicated solution if an easy a single functions. Keep the features short, centered, and easy to check. Use profiling equipment to seek out bottlenecks—locations where your code can take also long to operate or utilizes far too much memory.
Up coming, look at your databases queries. These frequently gradual factors down greater than the code by itself. Make sure Just about every query only asks for the information you truly need to have. Avoid Decide on *, which fetches everything, and alternatively select unique fields. Use indexes to speed up lookups. And prevent performing a lot of joins, especially across substantial tables.
In the event you observe the same info staying requested again and again, use caching. Shop the final results briefly working with resources like Redis or Memcached therefore you don’t have to repeat high-priced operations.
Also, batch your database operations when you can. As an alternative to updating a row one by one, update them in groups. This cuts down on overhead and can make your application a lot more economical.
Remember to exam with big datasets. Code and queries that operate good with one hundred data may well crash if they have to deal with one million.
To put it briefly, scalable apps are quick apps. Maintain your code restricted, your queries lean, and use caching when essential. These techniques assistance your software stay smooth and responsive, even as the load boosts.
Leverage Load Balancing and Caching
As your application grows, it's got to handle a lot more end users plus much more traffic. If everything goes via 1 server, it's going to speedily turn into a bottleneck. That’s wherever load balancing and caching can be found in. These two tools help keep your application speedy, secure, and scalable.
Load balancing spreads incoming website traffic throughout a number of servers. As an alternative to one particular server undertaking each of the operate, the load balancer routes end users to distinctive servers dependant on availability. What this means is no solitary server gets overloaded. If one server goes down, the load balancer can mail visitors to the Other individuals. Tools like Nginx, HAProxy, or cloud-centered alternatives from AWS and Google Cloud make this simple to setup.
Caching is about storing details quickly so it might be reused swiftly. When customers ask for precisely the same info all over again—like an item webpage or possibly a profile—you don’t have to fetch it within the databases each time. You could serve it from your cache.
There are two popular sorts of caching:
1. Server-facet caching (like Redis or Memcached) retailers details in memory for quickly obtain.
2. Shopper-aspect caching (like browser caching or CDN caching) suppliers static information near the user.
Caching minimizes databases load, improves pace, and will make your app additional effective.
Use caching for things which don’t alter generally. And usually ensure that your cache is updated when knowledge does alter.
Briefly, load balancing and caching are basic but impressive resources. Jointly, they help your app cope with more end users, continue to be quick, and Get well from complications. If you plan to increase, you would like each.
Use Cloud and Container Equipment
To build scalable programs, you require applications that let your app increase quickly. That’s where cloud platforms and containers come in. They provide you versatility, lower set up time, and make scaling A great deal smoother.
Cloud platforms like Amazon Internet Providers (AWS), Google Cloud System (GCP), and Microsoft Azure Enable you to rent servers and solutions as you require them. You don’t have to purchase hardware or guess long run ability. When targeted visitors increases, you are able to include a lot more assets with just a couple clicks or mechanically working with car-scaling. When targeted visitors drops, you'll be able to scale down to save cash.
These platforms also offer you expert services like managed databases, storage, load balancing, and protection applications. You can focus on building your app instead of managing infrastructure.
Containers are another vital Software. A container offers your app and every little thing it ought to operate—code, libraries, options—into 1 unit. This can make it effortless to move your app concerning environments, from the laptop computer towards the cloud, without surprises. Docker is the preferred Resource for this.
When your application works by using several containers, tools like Kubernetes assist you to manage them. Kubernetes handles deployment, scaling, and Restoration. If a single component within your app crashes, it restarts it automatically.
Containers also enable it to be very easy to separate portions of your app into products and services. You may update or scale elements independently, which happens to be great for overall performance and trustworthiness.
In brief, applying cloud and container applications suggests you'll be able to scale speedy, deploy simply, and Get better speedily when problems come about. If you want your app to mature with no restrictions, commence applying these equipment early. They help you save time, decrease chance, and help you remain focused on constructing, not correcting.
Keep track of Anything
If you don’t check your software, you received’t know when things go Mistaken. Checking helps you see how your app is undertaking, location problems early, and make greater conclusions as your application grows. It’s a important Portion of making scalable techniques.
Start out by monitoring basic metrics like CPU usage, memory, disk Area, and response time. These let you know how your servers and expert services are accomplishing. Tools like Prometheus, Grafana, Datadog, or New Relic will help you acquire and visualize this facts.
Don’t just observe your servers—observe your application too. Keep an eye on how long it will take for consumers to load webpages, how often problems happen, and where they happen. Logging resources like ELK Stack (Elasticsearch, Logstash, Kibana) or Loggly will help you see what’s taking place inside your code.
Set up alerts for essential issues. As an example, Should your response time goes over a limit or a company goes down, you'll want to get notified straight away. This allows you deal with difficulties rapidly, usually just before customers even recognize.
Monitoring can also be useful after you make improvements. In case you deploy a fresh function and find out a spike in glitches or slowdowns, you'll be able to roll it back right before it results in true injury.
As your application grows, website traffic and info increase. Without click here checking, you’ll skip indications of problems until it’s far too late. But with the correct equipment in place, you keep in control.
Briefly, monitoring can help you keep your application dependable and scalable. It’s not pretty much spotting failures—it’s about being familiar with your technique and making sure it really works well, even stressed.
Final Feelings
Scalability isn’t only for huge providers. Even tiny applications want a solid foundation. By coming up with cautiously, optimizing correctly, and using the suitable resources, you may build apps that mature smoothly with no breaking stressed. Commence smaller, think huge, and Establish intelligent.